Node RPC Access
You can access Node RPC directly from your Web3 Action functions. This allows you to send transactions on the Mainnet or testnets and read on-chain data automatically through Web3 Actions.
Setting up Web3 Actions to access the blockchain requires minimal setup — a single Javascript variable. No need to worry about copying/pasting RPC URLs, managing secret keys, etc. Tenderly takes care of all this for you in the background.
In this guide, you’ll learn how to configure your Web3 Action function code to use Tenderly’s production node to access the Mainnet.
Node RPC in Context
In Web3 Actions, access to Tenderly’s node is enabled through the context
object. Learn more about Web3 Actions Context here.
The context
object contains a property called gateways
which gives you access to the getGateway()
method. This method requires one argument Network
, which is used to specify the network you want to access.
The Network
argument is also an object which gives you access to all the supported networks in Node RPC:
Network.MAINNET
Network.GOERLI
Network.SEPOLIA
Network.HOLESKY
Network.POLYGON
Network.MUMBAI
Network.OPTIMISTIC
Network.OPTIMISTIC_GOERLI
Network.ARBITRUM_SEPOLIA
Network.BASE
Network.BASE_GOERLI
Network.BOBA_ETHEREUM
Network.BOBA_GOERLI
Network.BOBA_BINANCE
Network.BOBA_BINANCE_RIALTO
You can see the network list in @tenderly/actions npm package here.
To demonstrate how all of this comes together, here’s an example Javascript variable that is configured to access the Mainnet:
const defaultGatewayURL = context.gateways.getGateway(Network.MAINNET);
Example Web3 Action function to access the Mainnet
Let’s create a simple Web3 Action that will get triggered every time a new block is mined on the Mainnet. Each time a block gets mined, the Web3 Action will execute the custom code, also referred to as “function”. Read more about Web3 Action functions here.
For the sake of simplicity, the example function below will get the block number of the latest block mined and log it to the console.
You can read the comments to understand what each line of code does.
// Required libraries that must be included for the code to work
const ethers = require('ethers');
const { Network } = require('@tenderly/actions');
// Do not change the default 'actionFn' name.
const actionFn = async (context, blockEvent) => {
// Setting a variable that will store the Node RPC RPC URL and secret key
const defaultGatewayURL = context.gateways.getGateway(Network.MAINNET);
// Using the Ethere.js provider class to call the RPC URL
const provider = new ethers.providers.JsonRpcProvider(defaultGatewayURL);
// Logging the block number of the latest mined block
console.log(await provider.getBlockNumber());
};
// Exporting the default module. Do not change this.
module.exports = { actionFn };
Next, go to the Dashboard → Web3 Actions → Add Action.
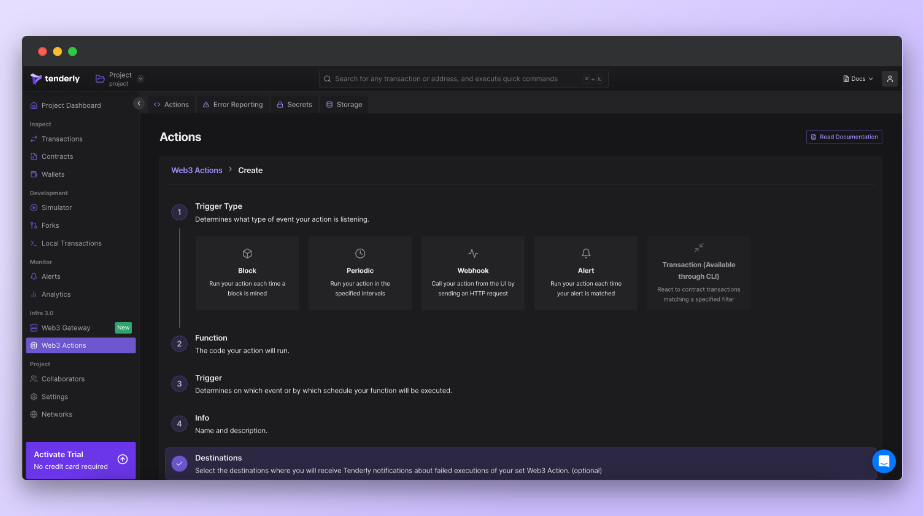
Web3 Action set up wizard
- For the Trigger Type, select Block.
- In the Function textbox, paste the code from the example above and click Next.
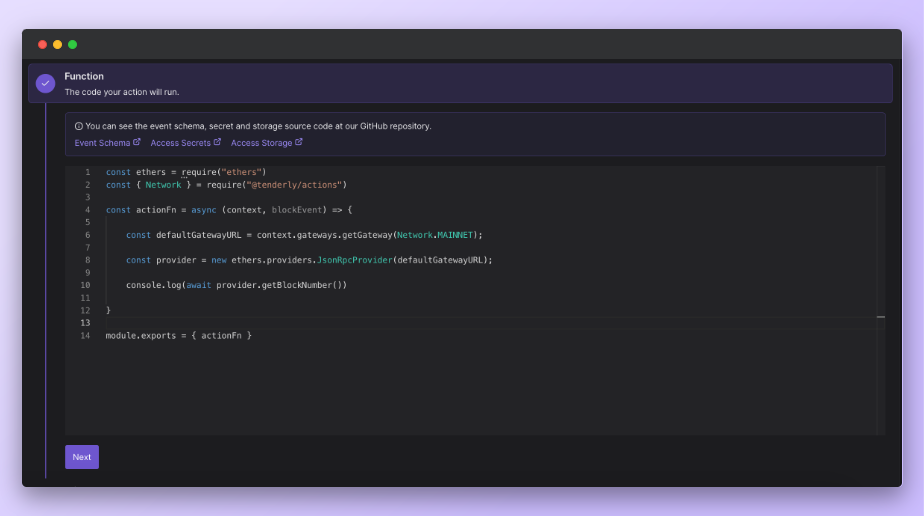
Custom code that is executed when the Web3 Action is triggered
- Set the Trigger by selecting the network. In our case, that is Mainnet.
- Also, set the Block Period to tell Tenderly how often to execute the Web3 Action function. For example, if you put 1, the Web3 Action will be executed once every block is mined.
- In the Info fields, add a name for the Web3 Action and an optional description.
- You can also set a Destination where you’ll receive notifications about failed executions of the Web3 Action.
- Lastly, click Create to deploy the Web3 Action.
From the Execution History page, we can see that the Web3 Action is being executed successfully.
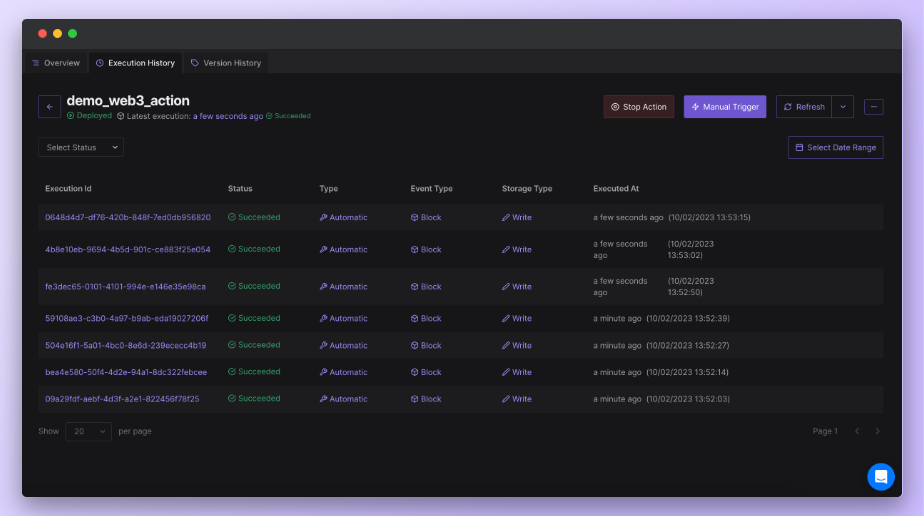
Web3 Action execution history
You can click on the Execution ID to see details about the executed Web3 Action and the payload in JSON.
Helpful resources
For more information on how to manage your Web3 Actions and monitor execution errors, read the following guides: